QSS
ウィンドウ
スタンドアロン
コードを表示
# -*- coding: utf-8 -*-
import sys
from PySide2 import QtWidgets
class MainWindow(QtWidgets.QMainWindow):
"""
メインウィンドウ
"""
def __init__(self, logger=None, parent=None):
"""
:param logger: ロガー
:type logger: logging.Logger
"""
super(MainWindow, self).__init__(parent)
self.logger = logger
self.setGeometry(650, 200, 1200, 900)
toolName = 'ツール名'
self.setObjectName(toolName)
self.setWindowTitle(toolName)
self.set_ui()
self.errorDialog = QtWidgets.QErrorMessage(self)
def set_ui(self):
"""
メインウィンドウの初期化
"""
wrapper = QtWidgets.QWidget()
self.setCentralWidget(wrapper)
# ルートのウィジェット
rootArea = QtWidgets.QVBoxLayout()
wrapper.setLayout(rootArea)
def launch(logger):
"""
メインウィンドウの起動
:param logger: ロガー
:type logger: logging.Logger
"""
app = QtWidgets.QApplication(sys.argv)
ui = MainWindow(logger=logger)
ui.show()
sys.exit(app.exec_())
python 2系 / 3系 互換 ウィンドウ
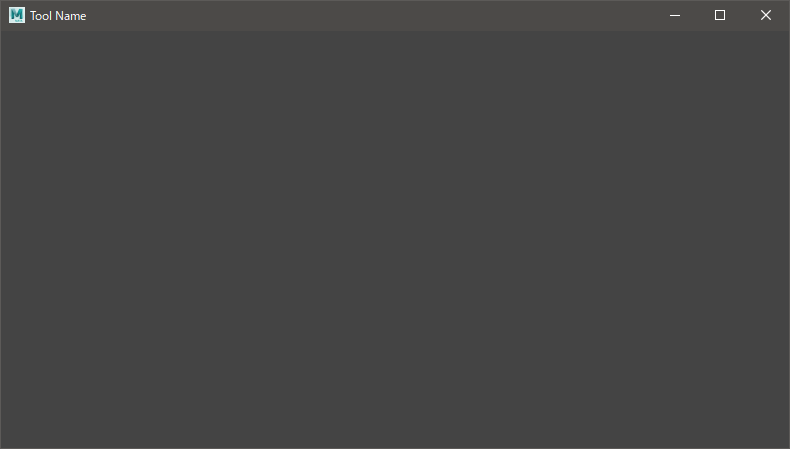
コードを表示
# -*- coding: utf-8 -*-
from __future__ import (absolute_import, division, print_function, unicode_literals)
import maya.cmds as cmds
from maya import OpenMayaUI
from PySide2.QtWidgets import *
from PySide2.QtGui import *
from PySide2.QtCore import *
from shiboken2 import wrapInstance
'''
設定項目
========================================================================================================================
'''
# ツール名の設定
toolName = 'Tool Name'
def get_maya_pointer():
OpenMayaUI.MQtUtil.mainWindow()
ptr = OpenMayaUI.MQtUtil.mainWindow()
widget = wrapInstance(int(ptr), QWidget)
return widget
'''
UI
========================================================================================================================
'''
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent)
# 起動時のウィンドウサイズ
self.setGeometry(650, 200, 1200, 900)
self.setObjectName(toolName)
self.setWindowTitle(toolName)
self._initUI()
self.errorDialog = QErrorMessage(self)
def _initUI(self):
wrapper = QWidget()
self.setCentralWidget(wrapper)
'''
####################################################################################################################
====================================================================================================================
UI
====================================================================================================================
####################################################################################################################
'''
# ルートのウィジェット
rootArea = QVBoxLayout()
wrapper.setLayout(rootArea)
'''
####################################################################################################################
====================================================================================================================
スロット(関数)
====================================================================================================================
####################################################################################################################
'''
def launch():
maya_win = get_maya_pointer()
ui = MainWindow(parent=maya_win)
ui.show()
return ui
# 起動 | ファイルで読み込む場合は消す
launch()
python 2系 / 3系 互換 フラットウィンドウ
コードを表示
# -*- coding: utf-8 -*-
"""
フラットウィンドウ
"""
from __future__ import (absolute_import, division, print_function, unicode_literals)
import sys
from PySide2 import QtWidgets, QtGui, QtCore
class MainWindow(QtWidgets.QMainWindow):
"""
フラットウィンドウ
"""
def mouseReleaseEvent(self, pos):
"""
マウスリリース時のイベント
"""
self.mc_x = pos.x()
self.mc_y = pos.y()
def mousePressEvent(self, pos):
"""
マウスプレス時のイベント
"""
self.mc_x = pos.x()
self.mc_y = pos.y()
def mouseMoveEvent(self, pos):
"""
マウスを移動させた際のイベント
"""
winX = pos.globalX() - self.mc_x
winY = pos.globalY() - self.mc_y
self.move(winX, winY)
# ------------------------------------------------------------------------------------------------------------------
#
#
# ウィンドウ設定
#
#
# ------------------------------------------------------------------------------------------------------------------
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent=parent)
self.bg_color_1 = '#1E73BE'
self.bg_color_2 = '#444'
self.mc_x = None
self.mc_y = None
window_width = 1000
window_height = 500
window_opacity = 0.8
# ウィンドウの透明度
self.setWindowOpacity(window_opacity)
# ウィンドウサイズ
self.resize(QtCore.QSize(window_width, window_height))
self.setWindowFlags(QtCore.Qt.Window | QtCore.Qt.FramelessWindowHint)
self.paletteUI()
wrapper = QtWidgets.QWidget()
self.setCentralWidget(wrapper)
# --------------------------------------------------------------------------------------------------------------
#
#
# UI
#
#
# --------------------------------------------------------------------------------------------------------------
root_Area = QtWidgets.QVBoxLayout()
wrapper.setLayout(root_Area)
close_Area = QtWidgets.QHBoxLayout()
root_Area.addLayout(close_Area)
close_Area.addStretch()
self.close_Btn = QtWidgets.QPushButton('close')
self.close_Btn.setFixedWidth(100)
self.close_Btn.clicked.connect(self.close_window)
close_Area.addWidget(self.close_Btn)
root_Area.addStretch()
def close_window(self):
"""
ウィンドウを閉じる
"""
self.close()
def paletteUI(self):
"""
角丸やウィンドウのカラーはここでいじる
"""
Palette = QtGui.QPalette()
# ウィンドウのバックカラー。色情報は UIに関する設定項目で設定済み
set_colors = [self.bg_color_1, self.bg_color_2]
# ↓topRight()をbottomRight()にかえると斜めにグラデ
gradient = QtGui.QLinearGradient(QtCore.QRectF(
self.rect()).topLeft(), QtCore.QRectF(self.rect()).topRight())
# グラデ
gradient.setColorAt(0.0, QtGui.QColor(set_colors[0]))
gradient.setColorAt(1.0, QtGui.QColor(set_colors[1]))
Palette.setBrush(QtGui.QPalette.Background, QtGui.QBrush(gradient))
self.setPalette(Palette)
# 角丸の設定
path = QtGui.QPainterPath()
path.addRoundedRect(self.rect(), 10, 10) # ここの数値で角丸の丸さが決まる
region = QtGui.QRegion(path.toFillPolygon().toPolygon())
self.setMask(region)
def launch():
"""
ウィンドウの起動
"""
standalone_app = QtWidgets.QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(standalone_app.exec_())
if __name__ == '__main__':
launch()
ウィンドウを画面中央へ移動させる
def center_window(self):
"""
ウィンドウをセンターへ移動
"""
centerPoint = QtGui.QScreen.availableGeometry(QtWidgets.QApplication.primaryScreen()).center()
fg = self.frameGeometry()
fg.moveCenter(centerPoint)
self.move(fg.topLeft())
#
# サイズと位置調整
#
width = 900
height = 500
# ディスプレイサイズ
app = QtWidgets.QApplication
geometry = app.primaryScreen().geometry()
frame_size = self.frameSize()
self.resize(width, height)
self.move(geometry.width() / 2 - frame_size.width() / 2, geometry.height() / 2 - frame_size.height() / 2)
QWidget
QWidget にスタイルシート貼れるようになるメソッド
下記をオーバーライドする。
def paintEvent(self, event):
opt = QtWidgets.QStyleOption()
opt.init(self)
painter = QtGui.QPainter(self)
style = self.style()
style.drawPrimitive(QtWidgets.QStyle.PE_Widget, opt, painter, self)
QPushButton
アイコン画像のセット
self.setIcon(QtGui.QIcon(self.icon_path))
self.setIconSize(QtCore.QSize(200,200))
入力系ウィジェット
基本的には QLineEdit と QPlainTetxtEdit の使い方は同じ。一行だけか長文使えるかの違い。
文字入力
QLineEdit
self.lineEdit = QLinedit()
# テキスト変更時のシグナル
self.lineEdit.textChanged.connect(self.test)
# テキストのセット
self.lineEdit.setText(text)
# テキストの内容の取得
plainText = self.lineEdit.text()
# 初期化
self.lineEdit.clear()
クリックして下さい
# -*- coding: utf-8 -*-
from __future__ import (absolute_import, division, print_function, unicode_literals)
import maya.cmds as cmds
from maya import OpenMayaUI
from PySide2.QtWidgets import *
from PySide2.QtGui import *
from PySide2.QtCore import *
from shiboken2 import wrapInstance
# ツール名の設定
toolName = 'QlineEdit'
def get_maya_pointer():
OpenMayaUI.MQtUtil.mainWindow()
ptr = OpenMayaUI.MQtUtil.mainWindow()
widget = wrapInstance(int(ptr), QWidget)
return widget
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent)
# 起動時のウィンドウサイズ
self.setGeometry(650, 200, 600, 300)
self.setObjectName(toolName)
self.setWindowTitle(toolName)
self._initUI()
self.errorDialog = QErrorMessage(self)
def _initUI(self):
wrapper = QWidget()
self.setCentralWidget(wrapper)
#===================================================================================================================
# UI 作成
#===================================================================================================================
# ルートのウィジェット
rootArea = QVBoxLayout()
wrapper.setLayout(rootArea)
# テキストエディットの設定
self.lineEdit = QLineEdit()
self.lineEdit.textChanged.connect(self.test_1) # テキスト変更時のシグナル
rootArea.addWidget(self.lineEdit)
# 変更不可 にする
#self.lineEdit.setReadOnly(True)
# プッシュボタン
self.test_1_Btn = QPushButton('内容をスクリプトエディタに表示')
self.test_1_Btn.clicked.connect(self.test_1) # クリック時のシグナル
rootArea.addWidget(self.test_1_Btn)
self.test_2_Btn = QPushButton('「テスト」の文字を記載')
self.test_2_Btn.clicked.connect(self.test_2) # クリック時のシグナル
rootArea.addWidget(self.test_2_Btn)
self.test_3_Btn = QPushButton('初期化')
self.test_3_Btn.clicked.connect(self.test_3) # クリック時のシグナル
rootArea.addWidget(self.test_3_Btn)
rootArea.addStretch()
#===================================================================================================================
# スロット
#===================================================================================================================
def test_1(self):
# QLineEdit に入力されている内容を取得
text = self.lineEdit.text()
print(text)
def test_2(self):
# lineEdit に任意の文字を記入
self.lineEdit.setText('テスト')
def test_3(self):
# 初期化
self.lineEdit.clear()
def launch():
maya_win = get_maya_pointer()
ui = MainWindow(parent=maya_win)
ui.show()
return ui
# 起動 | ファイルで読み込む場合は消す
launch()
QPlainTextEdit
self.plainTextEdit = QPlainTextEdit()
# テキスト変更時のシグナル
self.plainTextEdit.textChanged.connect(self.test)
# テキストのセット
self.plainTextEdit .setPlainText(text)
# テキストの内容の取得
plainText = self.plainTextEdit .toPlainText()
# 初期化
self.plainTextEdit.clear()
クリックして下さい
# -*- coding: utf-8 -*-
from __future__ import (absolute_import, division, print_function, unicode_literals)
import maya.cmds as cmds
from maya import OpenMayaUI
from PySide2.QtWidgets import *
from PySide2.QtGui import *
from PySide2.QtCore import *
from shiboken2 import wrapInstance
# ツール名の設定
toolName = 'QPlainTextEdit'
def get_maya_pointer():
OpenMayaUI.MQtUtil.mainWindow()
ptr = OpenMayaUI.MQtUtil.mainWindow()
widget = wrapInstance(int(ptr), QWidget)
return widget
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent)
# 起動時のウィンドウサイズ
self.setGeometry(650, 200, 600, 300)
self.setObjectName(toolName)
self.setWindowTitle(toolName)
self._initUI()
self.errorDialog = QErrorMessage(self)
def _initUI(self):
wrapper = QWidget()
self.setCentralWidget(wrapper)
#===================================================================================================================
# UI 作成
#===================================================================================================================
# ルートのウィジェット
rootArea = QVBoxLayout()
wrapper.setLayout(rootArea)
# テキストエディットの設定
self.plainTextEdit = QPlainTextEdit()
self.plainTextEdit.textChanged.connect(self.test_1) # テキスト変更時のシグナル
rootArea.addWidget(self.plainTextEdit)
# 変更不可
#self.plainTextEdit.setReadOnly(True)
# プッシュボタン
self.test_1_Btn = QPushButton('内容をスクリプトエディタに表示')
self.test_1_Btn.clicked.connect(self.test_1) # クリック時のシグナル
rootArea.addWidget(self.test_1_Btn)
self.test_2_Btn = QPushButton('「テスト」の文字を記載')
self.test_2_Btn.clicked.connect(self.test_2) # クリック時のシグナル
rootArea.addWidget(self.test_2_Btn)
self.test_3_Btn = QPushButton('初期化')
self.test_3_Btn.clicked.connect(self.test_3) # クリック時のシグナル
rootArea.addWidget(self.test_3_Btn)
#===================================================================================================================
# スロット
#===================================================================================================================
def test_1(self):
# plainText に入力されている内容を取得
plainText = self.plainTextEdit.toPlainText()
print(plainText)
def test_2(self):
# plainTextEdit に任意の文字を記入
self.plainTextEdit.setPlainText('テスト')
def test_3(self):
# 初期化
self.plainTextEdit.clear()
def launch():
maya_win = get_maya_pointer()
ui = MainWindow(parent=maya_win)
ui.show()
return ui
# 起動 | ファイルで読み込む場合は消す
launch()
数値入力
QSpinBox
spinBox = QSpinBox()
spinBox.setMinimum(0) # 最小値
spinBox.setMaximum(10) # 最大値
spinBox.setSuffix("min") # 接尾辞を設定
spinBox.setSingleStep(10) # ステップ数
QDoubleSpinBox
# 小数点以下の桁数を設定
self.doubleSpinBox.setDecimals()
QSlider
modo10のスクリプトについて調べてみた その16
入力切替
QCheckBox
# チェックボックスの設置
self.hoge_CheckBox = QCheckBox('hoge')
# チェックボックスのオン/オフ
self.hoge_CheckBox.setChecked(True)
self.hoge_CheckBox.setChecked(False)
# 状態変更時 のシグナル
self.hoge_CheckBox.toggled.connect(self.slot)
# チェックボックスのオン/オフの取得
self.hoge_CheckBox.isChecked()
QRadioBox
# チェックが入っているID取得
hogeID = self.hoge_BtnGroup.checkedId()
# グループ化
self.group_A = QButtonGroup()
self.group_A.addButton(self.radio_a,1)
self.group_A.addButton(self.radio_b,2)
【PySide】横レイアウト/ラジオボタン/仕切り
QComboBox
self.comboBox = QComboBox()
# 複数入れる場合はリストをそのまま突っ込めば入る
self.comboBox.addItems(text_list) # コンボボックスに入力
# テキスト検索にして setInsertPolicy を設定しない場合、
# 入力された文字列がそのままコンボボックスに入る
self.comboBox.setEditable(True) # テキスト検索 ON
self.comboBox.setInsertPolicy(QComboBox.NoInsert) # テキスト追加 OFF
self.comboBox.currentIndex() # コンボボックスのインデックス取得
# 完全一致のアイテム名のインデックスを取得
self.comboBox.findText(current_combo_text, QtCore.Qt.MatchFixedString)
# シグナル
#======================================================================
self.comboBox.currentIndexChanged.connect(slot) # 変更時
# スロット
#======================================================================
self.comboBox.setCurrentIndex(index) # インデックスで選択項目指定
表示系ウィジェット
QLabel
画像を表示する
self.icon_path = 'D:\\hoge\\hoge.png'
self.icon_width = 50
self.icon_height = 50
self.icon_Lbl = QtWidgets.QLabel()
self.icon_Lbl.setAlignment(Qt.AlignCenter) # 中央へ整列
pixmap = QtGui.QPixmap(self.icon_path) # pixmap の作成
pixmap = pixmap.scaled(
self.icon_width, self.icon_height,
QtCore.Qt.KeepAspectRatio) # アイコンのサイズ
self.icon_Lbl.setPixmap(pixmap) # pixmap の設置
self.icon_Area.addWidget(self.icon_Lbl) # ウィジェット の設置
QListWidget
クリックして下さい
# リストウィジェットの作成
self.listWidget= QListWidget()
# リストを空にする
self.listWidget.clear()
# リストに項目を追加する
self.listWidget(['A', 'B', 'C'])
# リストへ変数「item」を追加する
self.listWidget.addItem(item)
# 渡した数字の列数を選択する。-1 で選択解除
self.listWidget.setCurrentRow(-1)
# 指定した行数のアイテムを選択する 複数可能
listWidget.item(row).setSelected(True)
# アイコン付き
widgetItem = QListWidgetItem(item)
widgetItem.setIcon(QIcon(iconPath))
targetlistWidget.addItem(widgetItem)
'''
オプション項目
'''
# 複数選択を可能にする
self.listWidget.setSelectionMode(QAbstractItemView.MultiSelection)
# よく使う複数選択方法
self.listWidget.setSelectionMode(QAbstractItemView.ExtendedSelection)
# アイテムにアイコンを付けてリストへ入れる
item = QListWidgetItem(targetItem) # 目的のものをアイテム化
item.setIcon(QIcon(":\\mayaIcon.png")) # アイコンセット
self.listWidget.addItem(item) # アイテムをリストに追加
# フォーカスを外す
self.listWidget.clearFocus()
'''
シグナル
'''
# 選択されているものが変更された場合のシグナル
self.listWidget.itemSelectionChanged.connect(selectChange_Func)
# アイテムシングルクリック
self.listWidget.itemClicked.connect()
# アイテムダブルクリック
self.listWidget.itemDoubleClicked.connect()
'''
取得
'''
# 指定した行のアイテムが選択されているかどうか
item = listWidget.item(row)
print item.isSelected()
# 選択されている項目の取得
# ↓ のコードにテキストや行などの情報が格納される
slctItems = self.treeWidget.selectedItems()
# 選択されている項目の文字列を取得
slctItemsText = self.listWidget.selectedItems()[0].text()
# 選択されている項目の文字列を取得 単体の選択時
slctItem_text = self.listWidget.currentItem().text()
# 選択されている項目の列数を取得
slctItemIndex = self.listWidget.currentRow()
# text 完全一致の名前の項目がある場合にその行を返す
find_items= self.listWidget.findItems('test', Qt.MatchExactly)
if len(find_items) > 0:
print(self.listWidget.row(items[0]))
if len(find_items) > 0:
row = root_listWidget.row(find_items[0])
self.listWidget.setCurrentRow(row)
# https://stackoverflow.com/questions/24433357/how-can-i-get-qlistwidget-item-by-nam
# 全項目の取得
item_list = []
count = listWidget.count()
for row in range(count):
text = listWidget.item(row).text() # 行数のアイテム名取得
item_list.append(text)
# 全項目の選択
count = pfb_listWidget.count()
for row in range(count):
pfb_listWidget.item(row).setSelected(True)
QTableWidget
カラム幅について
クリックして下さい
# 列 colmun
#----------------------------------------------------------------------
# 列数の設定
tableWidget.setColumnCount(col_count)
# 列の項目名の設置
col_01 = QTableWidgetItem('項目名')
tableWidget.setHorizontalHeaderItem(0, col_01)
# 列のカラム幅を固定する
header = tableWidget.horizontalHeader()
for i in range(col_count):
header.setSectionResizeMode(i, QHeaderView.ResizeToContents)
# 一括で行う カラム 設定
#---------------------------------------------------------------------------------------------------------------
col_count = 3
tableWidget.setColumnCount(col_count)
col_content_list = ['Unity', 'YAML', ' ']
header = tableWidget.horizontalHeader()
for i in range(col_count):
header.setSectionResizeMode(i, QHeaderView.ResizeToContents)
item = QTableWidgetItem(col_content_list[i])
tableWidget.setHorizontalHeaderItem(i, item)
# 行 row
#----------------------------------------------------------------------
# 行数の設定
tableWidget.setRowCount(row_count)
# 一括で行う ロウ 設定
#---------------------------------------------------------------------------------------------------------------
row_count = 9
tableWidget.setRowCount(row_count)
row_content_list = [
'名前',
'Layer',
'Static',
'Light Probes',
'Refrection Probes',
'Cast Shadows',
'Recieve Shadows',
'Motion Vectors',
'Materials : Size',
'Dynamic Occluded'
]
for i in range(row_count):
item = QTableWidgetItem(' ' + row_content_list[i])
tableWidget.setVerticalHeaderItem(i, item)
# 追加
#----------------------------------------------------------------------
item = QTableWidgetItem(item_name)
tableWidget.setItem(row, col, item )
ウィジェット
フォント
hoge.setFont(QtGui.QFont('游ゴシック'))
hoge.setFont(QtGui.QFont('Arial'))
整列
hoge.setAlignment(Qt.AlignCenter)
hoge.setAlignment(Qt.AlignRight)
その他
# レイアウトに設置されているウィジェットを削除
self.qlayout.removeWidget(self.widget)
del self.widget
# QWidget 直下のレイアウトの余白を削除
self.root_area.setContentsMargins(0, 0, 0, 0)